Revise Revise Revise

Embarking on Your Python Journey: A Comprehensive Guide for Beginners

Python is a versatile and powerful programming language that has gained immense popularity over the years. Whether you’re a complete beginner or an experienced programmer looking to explore a new language, Python offers a gentle learning curve and a wide range of applications. In this comprehensive guide, we’ll take you through the essential steps to get started with Python, covering everything from installation to writing your first program. So, let’s dive in and unlock the world of Python programming!
Understanding Python and Its Benefits

Before we begin, it’s important to grasp the fundamentals of Python and why it has become a favorite among developers. Python is an interpreted, high-level programming language known for its simplicity, readability, and versatility. Here’s why Python is an excellent choice for beginners:
- Simplicity and Readability: Python’s syntax is designed to be easy to understand and write, even for those new to programming. Its clean and structured code makes it ideal for learning the basics.
- Versatility: Python can be used for a wide range of tasks, including web development, data analysis, machine learning, automation, and more. Its versatility allows you to explore various domains.
- Large Community and Support: Python has a vast and active community, which means you’ll find extensive documentation, tutorials, and support online. This makes it easier to learn and troubleshoot.
- Extensive Libraries and Frameworks: Python boasts a vast ecosystem of libraries and frameworks, such as NumPy, Pandas, Django, and Flask, which simplify complex tasks and accelerate development.
- Cross-Platform Compatibility: Python runs on multiple platforms, including Windows, macOS, and Linux, making it accessible and convenient for developers.
Setting Up Your Python Environment

To start your Python journey, you’ll need to set up your development environment. Here’s a step-by-step guide:
Step 1: Download and Install Python:
- Visit the official Python website at https://www.python.org/downloads/ and download the latest version of Python.
- Choose the installer that matches your operating system (Windows, macOS, or Linux).
- Follow the installation instructions provided by the installer.
Step 2: Verify Python Installation:
- Open your terminal or command prompt.
- Type
python --version
(orpython3 --version
if you have multiple Python versions) and press Enter. - If Python is installed correctly, you should see the version number displayed.
Step 3: Install Integrated Development Environment (IDE):
- An IDE provides a user-friendly interface for writing and debugging code. Some popular Python IDEs include PyCharm, Visual Studio Code, and Spyder.
- Download and install your preferred IDE.
- Set up your project and configure the Python interpreter within the IDE.
Writing Your First Python Program

Now that your environment is set up, it’s time to write your first Python program! Here’s a simple “Hello, World!” program to get you started:
# This is a comment in Python
# Print a message
print("Hello, World!")
# Perform a simple calculation
result = 5 + 3
print("The result is:", result)
To run this program:
- Open your IDE.
- Create a new Python file (e.g.,
hello_world.py
). - Copy and paste the code into the file.
- Save the file.
- Run the program by clicking the “Run” button or using the appropriate keyboard shortcut.
- The output will be displayed in the console or the output panel of your IDE.
Basic Python Syntax and Concepts

Python follows a set of rules and conventions known as syntax. Understanding these fundamentals is crucial for writing correct and efficient code. Here are some key concepts:
- Indentation: Python uses indentation (whitespace) to define code blocks. Proper indentation is essential for the correct execution of your programs.
- Variables: Variables are used to store data in Python. You can assign values to variables using the
=
operator. For example:name = "John"
. - Data Types: Python supports various data types, including integers (
int
), floating-point numbers (float
), strings (str
), lists (list
), dictionaries (dict
), and more. - Operators: Python provides a range of operators for performing arithmetic, comparison, logical, and assignment operations.
- Comments: Comments are used to add explanations or notes to your code. They are ignored by the interpreter and start with a
#
symbol.
Exploring Python’s Built-in Functions

Python comes with a rich set of built-in functions that simplify common tasks. Here are some commonly used functions:
- Input Function: The
input()
function allows you to accept user input. It waits for the user to enter data and returns it as a string. - Type Conversion: You can convert data types using functions like
int()
,float()
, andstr()
. For example:int_value = int("123")
. - String Manipulation: Python offers various string methods for tasks like concatenation, slicing, and formatting.
- Mathematical Functions: Python provides mathematical functions like
abs()
,pow()
, andround()
for performing calculations. - Conditional Statements: Use
if
,elif
, andelse
statements to control the flow of your program based on conditions.
Working with Lists and Dictionaries

Python provides two powerful data structures: lists and dictionaries.
- Lists: Lists are ordered collections of elements, which can be of different data types. You can access elements using indexing and perform various operations like appending, removing, and sorting.
fruits = ["apple", "banana", "orange"]
print(fruits[1]) # Output: banana
fruits.append("grape")
print(fruits) # Output: ["apple", "banana", "orange", "grape"]
- Dictionaries: Dictionaries store key-value pairs, allowing efficient data retrieval. Keys must be unique, and values can be of any data type.
student = {"name": "Alice", "age": 20, "grades": [85, 92, 78]}
print(student["name"]) # Output: Alice
print(student["grades"][1]) # Output: 92
Control Flow and Loops
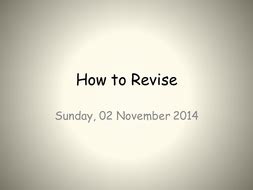
Python offers control flow statements and loops to manage the execution of your code:
- if-else Statements: Use
if
andelse
to execute different blocks of code based on conditions. - for Loops:
for
loops iterate over a sequence (e.g., a list) and execute a block of code for each element. - while Loops:
while
loops repeatedly execute a block of code as long as a condition is true.
Function Definition and Calling

Functions are reusable blocks of code that perform specific tasks. Here’s how to define and call functions in Python:
def greet(name):
print(f"Hello, {name}!")
# Calling the function
greet("Alice") # Output: Hello, Alice!
Handling Errors and Exceptions

Python provides mechanisms to handle errors and exceptions, ensuring your programs can gracefully manage unexpected situations:
- try-except Blocks: Use
try
to execute a block of code andexcept
to catch and handle exceptions. - Exception Types: Python has various exception types, such as
ValueError
,TypeError
, andIndexError
, which you can catch and handle specifically.
Exploring Python Libraries and Packages
Python’s strength lies in its extensive library ecosystem. Here are some popular libraries:
- NumPy: A powerful library for numerical computations and data analysis.
- Pandas: Offers data structures and data analysis tools for working with structured data.
- Matplotlib: A popular library for creating visualizations and plots.
- Scikit-learn: Provides machine learning algorithms and tools for data modeling.
- Django and Flask: Web frameworks for building web applications.
Best Practices and Tips for Python Programming
To become a proficient Python programmer, consider the following best practices:
- Code Readability: Write clean and readable code with proper indentation and meaningful variable names.
- Documentation: Document your code using comments and docstrings to explain its purpose and functionality.
- Modularization: Break down complex programs into smaller, reusable functions and modules.
- Error Handling: Implement proper error handling to ensure your programs can recover from errors gracefully.
- Version Control: Use version control systems like Git to track changes and collaborate effectively.
Conclusion
Python is an incredible programming language that empowers beginners and experienced developers alike. With its simplicity, versatility, and extensive community support, Python opens doors to a wide range of opportunities. By following this comprehensive guide, you’ve taken the first steps towards mastering Python. Continue exploring, practicing, and experimenting with Python to unlock its full potential. Happy coding!
FAQ
What is the best way to learn Python as a beginner?
+As a beginner, it’s recommended to start with interactive tutorials and online courses. Websites like Codecademy, Udemy, and Coursera offer comprehensive Python courses for beginners. Additionally, practicing with small projects and exploring Python’s official documentation will enhance your learning.
Is Python suitable for web development?
+Absolutely! Python is widely used for web development. Popular web frameworks like Django and Flask provide powerful tools and libraries for building web applications. Python’s simplicity and extensive libraries make it an excellent choice for web development projects.
Can I use Python for data science and machine learning?
+Yes, Python is a go-to language for data science and machine learning. Libraries like NumPy, Pandas, and Scikit-learn offer advanced data analysis and machine learning capabilities. Python’s versatility and robust community support make it an ideal choice for data-related tasks.
What are some popular Python IDEs for beginners?
+For beginners, PyCharm Community Edition, Visual Studio Code, and Spyder are excellent choices. These IDEs provide user-friendly interfaces, code completion, and debugging tools, making them perfect for learning and developing Python programs.
How can I contribute to the Python community?
+Contributing to the Python community is a great way to give back and enhance your skills. You can start by participating in open-source projects on platforms like GitHub, writing blog posts or tutorials, attending Python meetups, and sharing your knowledge with others.