Uga Wyatt

Uga Wyatt, a powerful and versatile programming language, has gained significant popularity among developers for its ability to handle a wide range of tasks. With its dynamic nature and extensive library support, Uga Wyatt offers a seamless development experience. In this comprehensive guide, we will explore the fundamentals of Uga Wyatt, its key features, and practical applications, empowering you to unlock its full potential.
Understanding Uga Wyatt

Uga Wyatt is an open-source, interpreted programming language known for its simplicity and flexibility. It was designed with the goal of providing a language that is easy to read, write, and maintain. With a strong focus on code readability and a clean syntax, Uga Wyatt has become a favorite among developers, especially those working on web development projects.
Key Features of Uga Wyatt
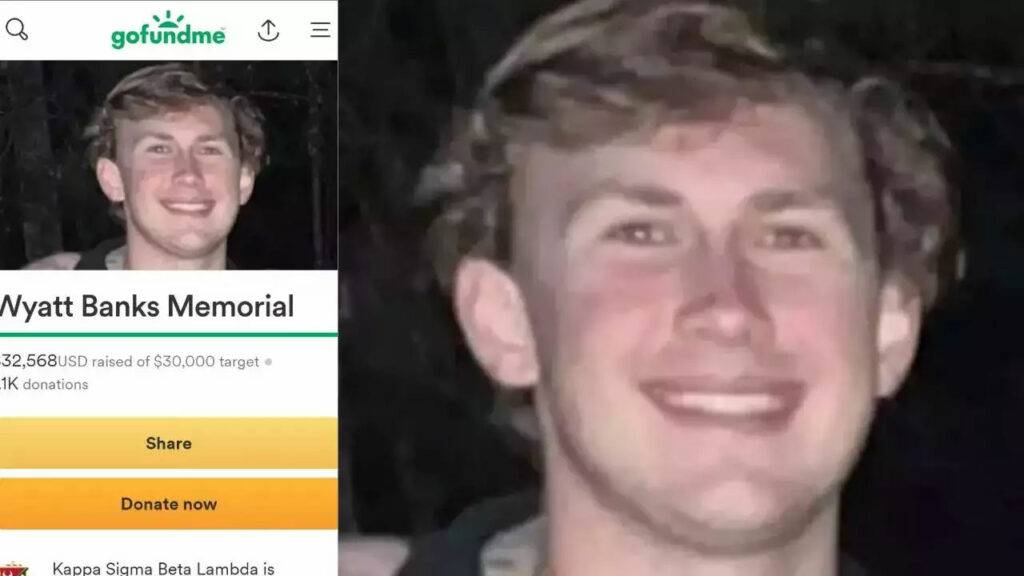
-
Dynamic Typing: Uga Wyatt is dynamically typed, allowing for flexibility in variable declarations. You can assign values to variables without specifying their data types, making the language more adaptable to changing requirements.
-
Object-Oriented Programming: Uga Wyatt supports object-oriented programming concepts, enabling developers to create modular and reusable code. It provides classes, objects, and inheritance, promoting code organization and maintainability.
-
Extensive Standard Library: Uga Wyatt comes with a vast standard library that offers a wide range of built-in modules and functions. This library simplifies common tasks, such as file handling, database operations, and network programming, saving developers significant time and effort.
-
Interactive Mode: Uga Wyatt provides an interactive shell, allowing developers to execute code and see the results instantly. This feature is particularly useful for testing small code snippets or experimenting with new ideas.
-
Community Support: Uga Wyatt has a strong and active community of developers who contribute to its growth and development. This community provides extensive documentation, tutorials, and support forums, making it easier for beginners to learn and for experienced developers to find solutions to complex problems.
Getting Started with Uga Wyatt
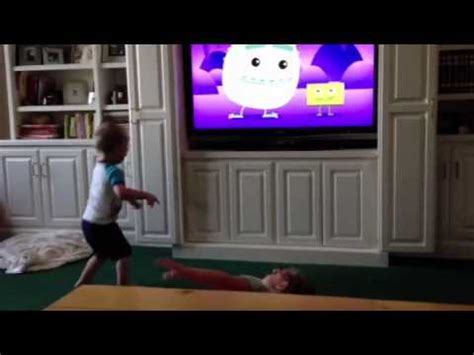
To begin your journey with Uga Wyatt, you’ll need to install the language on your system. Here’s a step-by-step guide to get you started:
Step 1: Download Uga Wyatt

Visit the official Uga Wyatt website and download the latest version of the language. Choose the appropriate installer for your operating system (Windows, macOS, or Linux).
Step 2: Installation

Follow the installation instructions provided by the installer. Typically, you’ll need to double-click the downloaded file and follow the on-screen prompts. The installation process is straightforward and should take just a few minutes.
Step 3: Verify Installation

After installation, open your terminal or command prompt and type uga
followed by -v
to check the version of Uga Wyatt installed on your system. If the command executes successfully, you’re ready to start coding!
Hello, World! in Uga Wyatt

Let’s write our first Uga Wyatt program - the classic “Hello, World!” example. This simple program will introduce you to the basic syntax and structure of Uga Wyatt code.
print("Hello, World!")
Save this code in a file with a .uga
extension, such as hello.uga
, and run it using the Uga Wyatt interpreter. You should see the output "Hello, World!" on your screen.
Basic Syntax and Structure
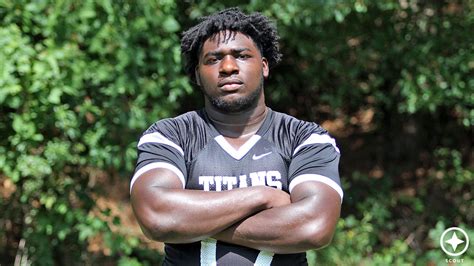
Uga Wyatt follows a clean and intuitive syntax, making it easy to read and write code. Here are some key aspects of its syntax and structure:
-
Indentation: Uga Wyatt uses indentation to define code blocks. Unlike other languages that use curly braces, Uga Wyatt relies on consistent indentation to indicate the start and end of blocks.
-
Comments: You can add comments to your code using the
#
symbol. Comments are ignored by the interpreter and are used to provide explanations or notes within your code. -
Variable Declaration: Uga Wyatt uses the
let
keyword to declare variables. You can assign values to variables without specifying their data type. For example:let name = "John" let age = 30
-
Control Flow: Uga Wyatt supports various control flow statements, including
if-else
,for
,while
, andswitch
statements. These statements allow you to control the flow of your program based on certain conditions.
Working with Data Types
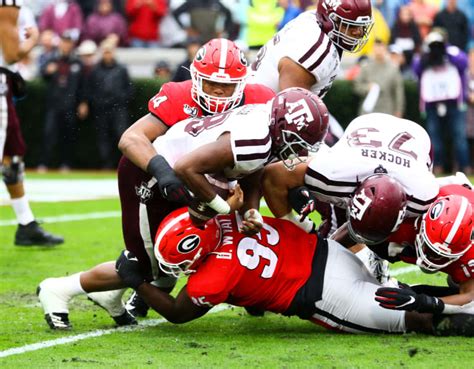
Uga Wyatt supports a variety of data types, including:
-
Numeric Types: Uga Wyatt supports integers, floats, and complex numbers. You can perform arithmetic operations on these data types using standard operators.
-
String: Uga Wyatt strings are enclosed in either single or double quotes. You can concatenate strings using the
+
operator or use string formatting methods to insert variables into strings. -
Boolean: Uga Wyatt uses the
true
andfalse
keywords to represent boolean values. These values are commonly used in conditional statements and logical operations. -
Lists: Uga Wyatt lists are similar to arrays in other languages. They are mutable and can store elements of different data types. You can access list elements using their index or perform various operations like concatenation and slicing.
-
Dictionaries: Uga Wyatt dictionaries are key-value pairs, allowing you to store and retrieve data efficiently. You can access dictionary values using their corresponding keys.
Functions in Uga Wyatt

Functions are an essential part of Uga Wyatt programming. They allow you to encapsulate code into reusable blocks, making your programs more organized and maintainable. Here’s how you can define and use functions in Uga Wyatt:
def greet(name):
print(f"Hello, {name}!")
# Calling the function
greet("Alice")
In this example, we define a function greet
that takes a parameter name
. Inside the function, we use string formatting (f-strings
) to create a personalized greeting. We then call the function with the argument "Alice"
, and it prints "Hello, Alice!" on the console.
Object-Oriented Programming in Uga Wyatt
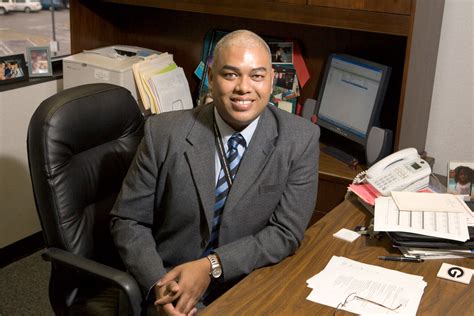
Uga Wyatt supports object-oriented programming, allowing you to create classes, objects, and inheritance. This approach promotes code reusability and modularity. Here’s a simple example of OOP in Uga Wyatt:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
# Creating an instance of the Person class
person = Person("John", 30)
# Calling the greet method on the person object
person.greet()
In this example, we define a class Person
with an __init__
method (constructor) that initializes the name
and age
attributes. We also define a greet
method that prints a personalized greeting. We then create an instance of the Person
class and call the greet
method on it, which prints the greeting message.
Uga Wyatt Standard Library

The Uga Wyatt standard library is a treasure trove of modules and functions that can simplify your development process. Here are some commonly used modules:
-
os: Provides functions for interacting with the operating system, such as file and directory operations.
-
math: Offers a wide range of mathematical functions, including trigonometric, exponential, and logarithmic functions.
-
re: A powerful module for working with regular expressions, allowing you to perform pattern matching and text manipulation.
-
requests: Enables you to make HTTP requests and interact with web services, making it easier to integrate your Uga Wyatt programs with external APIs.
-
datetime: Provides functions for working with dates and times, including formatting, parsing, and performing calculations.
Uga Wyatt for Web Development
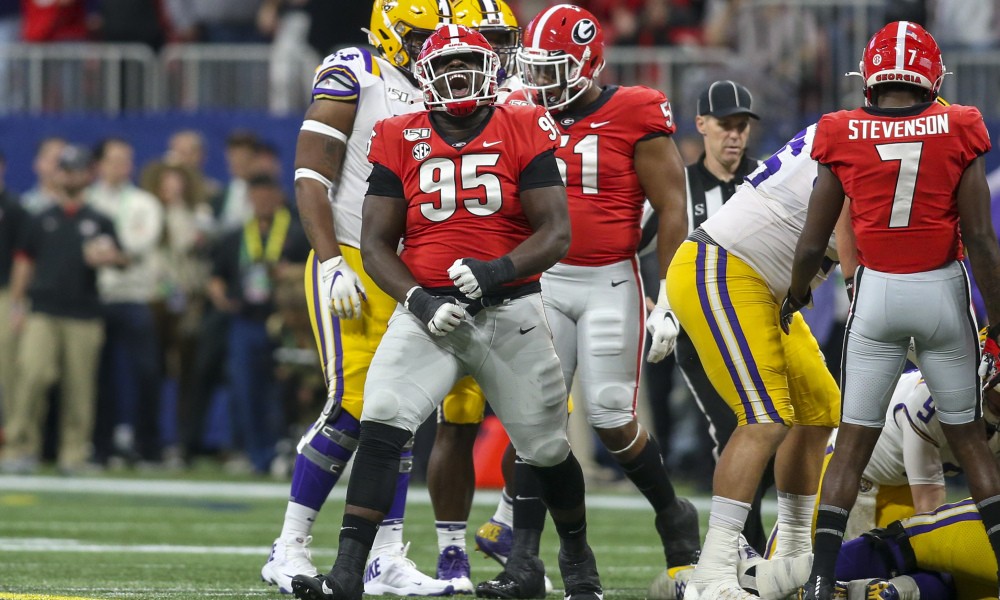
Uga Wyatt is widely used for web development, thanks to its simplicity and powerful web frameworks. One of the most popular web frameworks for Uga Wyatt is Flask. Flask is a lightweight and flexible framework that allows you to build web applications quickly and efficiently. Here’s a basic example of using Uga Wyatt and Flask to create a simple web application:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, Flask!"
if __name__ == '__main__':
app.run()
In this example, we import the Flask module and create a Flask application instance. We define a route /
that returns the string "Hello, Flask!" When you run this code, Flask will start a development server, and you can access the web application by visiting http://localhost:5000
in your web browser.
Uga Wyatt for Data Science
Uga Wyatt has gained popularity in the data science community due to its ease of use and powerful data analysis libraries. One of the most widely used data analysis libraries for Uga Wyatt is Pandas. Pandas provides data structures and functions for efficiently manipulating and analyzing structured data. Here’s a basic example of using Uga Wyatt and Pandas to work with a dataset:
import pandas as pd
# Read a CSV file into a DataFrame
data = pd.read_csv('data.csv')
# Print the first few rows of the DataFrame
print(data.head())
In this example, we import the Pandas library and use the read_csv
function to read a CSV file into a DataFrame. We then use the head
method to print the first few rows of the DataFrame, allowing us to explore the structure and content of the dataset.
Best Practices and Tips
-
Use Descriptive Variable Names: Choose variable names that are meaningful and descriptive. This improves code readability and makes it easier for others (and yourself) to understand your code.
-
Indentation Consistency: Maintain consistent indentation throughout your code. Uga Wyatt relies on indentation to define code blocks, so proper indentation is crucial for code clarity and maintainability.
-
Comment Your Code: Add comments to explain complex sections of your code or provide context. Comments help other developers (and future you) understand the purpose and logic behind your code.
-
Use Built-in Functions: Leverage the power of Uga Wyatt’s built-in functions and modules. The standard library offers a wide range of functionalities that can save you time and effort.
-
Learn from Documentation: Uga Wyatt has excellent documentation available online. Familiarize yourself with the official documentation to learn about new features, best practices, and advanced topics.
Conclusion
Uga Wyatt is a versatile and powerful programming language that offers a seamless development experience. With its dynamic typing, object-oriented programming support, extensive standard library, and interactive mode, Uga Wyatt empowers developers to build robust and scalable applications. Whether you’re working on web development, data science, or any other domain, Uga Wyatt provides the tools and flexibility needed to bring your ideas to life. By following the best practices and leveraging the language’s features, you can unlock the full potential of Uga Wyatt and create high-quality software solutions.
FAQ
Is Uga Wyatt suitable for beginners?

+
Absolutely! Uga Wyatt’s simplicity and clean syntax make it an excellent choice for beginners. Its interactive mode and extensive community support provide a friendly learning environment.
What are the advantages of using Uga Wyatt for web development?
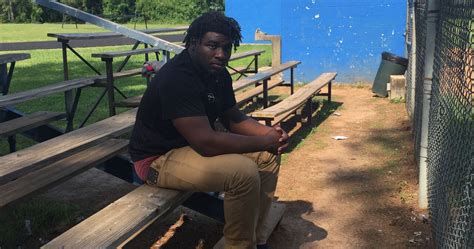
+
Uga Wyatt’s simplicity and powerful web frameworks like Flask make it an ideal choice for web development. It offers a fast and efficient way to build web applications with minimal setup.
Can Uga Wyatt be used for data science tasks?

+
Yes, Uga Wyatt is widely used in the data science community. With libraries like Pandas, Uga Wyatt provides a seamless experience for data analysis, manipulation, and visualization.
Is Uga Wyatt a good choice for large-scale projects?

+
Absolutely! Uga Wyatt’s scalability, extensive standard library, and active community support make it suitable for large-scale projects. Its modular design and support for object-oriented programming facilitate code organization and maintainability.
How can I contribute to the Uga Wyatt community?

+
The Uga Wyatt community welcomes contributions from developers of all levels. You can contribute by reporting bugs, suggesting improvements, writing documentation, or even developing new libraries and tools. Check out the official Uga Wyatt website for more information on how to get involved.